How to fetch data from API in flutter ?
Many apps needs fetch data from server and provide fresh data to users. There are different ways to achieve this in app. In this article we will learn how to fetch data from API in flutter.
Flutter provides http package to make http calls. In this article we will do a simple example of fetching data from Restful Api and display data in our screen.
If you are an android developer then you must know that if we use any library we have to add this library in build.gradle file and then sync the project. Same here if we have to use any library we have to add this in pubspec.yml file and sync the project. In Flutter to sync the project we use this command.
Lets start work
Create a new flutter project.
Add http library in pubspec.yaml
dependencies:
flutter:
sdk: flutter
http: ^0.13.4
This http library will be used to make http calls. In this example it will be used to call restful API and fetch data.
Rest API
https://gorest.co.in/public/v1/users
We will use this API in this example. It will return the users list that contains user’s id, name, email, gender and status.
{
"data": [
{
"id": 1644,
"name": "Andrzej Nowak",
"email": "andrzej.nowak1@gmail.com",
"gender": "male",
"status": "active"
},
{
"id": 1645,
"name": "Andrzej Nowak",
"email": "andrzej.nowak1432@gmail.com",
"gender": "male",
"status": "active"
},
{
"id": 1668,
"name": "T Rmakrishna",
"email": "hi@gmail.com",
"gender": "male",
"status": "active"
}
]
}
Create User Model Class
First of all create user model class that will contain the attributes of user.
class User {
int id;
String name;
String email;
String gender;
User(
{required this.id,
required this.name,
required this.email,
required this.gender});
factory User.fromJson(Map<String, dynamic> json) {
return User(
id: json['id'],
name: json['name'],
email: json['email'],
gender: json['gender']);
}
}
This is the user model class with its attributes. This class contains a method User.fromJson(). This method converts the json response into the user object.
Fetch Data
Now in main.dart create a stateful widget FirstScreen. And in this widget create a method fetchUsers().
Future<List<User>> fetchUsers() async {
var response = await http.get(Uri.parse(apiUrl));
return (json.decode(response.body)['data'] as List)
.map((e) => User.fromJson(e))
.toList();
}
This method make a get request to restful API and fetch data from server. And then decode the response into the list of users. Now we will display this users list in listview.
FutureBuilder is used to wait for response of http call and on response it will notified and will display data in listview.
Here is the complete code of this example
main.dart
class FirstScreen extends StatefulWidget {
const FirstScreen({Key? key}) : super(key: key);
@override
_FirstScreenState createState() => _FirstScreenState();
}
class _FirstScreenState extends State<FirstScreen> {
final String apiUrl = "https://gorest.co.in/public/v1/users";
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('First Screen'),
),
body: Container(
color: Colors.grey,
padding: const EdgeInsets.all(8),
child: FutureBuilder<List<User>>(
future: fetchUsers(),
builder: (context, snapshot) {
if (snapshot.hasData) {
List<User> users = snapshot.data as List<User>;
return ListView.builder(
itemCount: users.length,
itemBuilder: (context, index) {
return Container(
margin: EdgeInsets.all(8),
padding: EdgeInsets.all(8),
color: Colors.white,
child: Column(
children: [
Text(users[index].name),
Text(users[index].email),
Text(users[index].gender),
],
),
);
});
}
if (snapshot.hasError) {
print(snapshot.error.toString());
return Text('error');
}
return CircularProgressIndicator();
},
),
));
}
Future<List<User>> fetchUsers() async {
var response = await http.get(Uri.parse(apiUrl));
return (json.decode(response.body)['data'] as List)
.map((e) => User.fromJson(e))
.toList();
}
}
Output
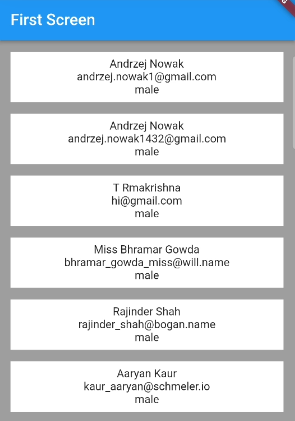
This was a simple example of http get request in Flutter with simple Json data. Json is not very complex.
Conclusion
In this tutorial we have learned that how to fetch data from Api in flutter using http package. Here we fetch a simple Json from server and parse it to model class. Hope this will help you to make easy your projects.
Thank you for Reading…