How to create popup menu item in flutter
Popup menu item in flutter is an overflow menu like in android and ios .It is one of the most used widget in any type of apps. It is used to show multiple actions/options to users. In this article we will learn how to create popup menu item in flutter.
Let’s start work.
Flutter Popup menu button Example
It takes some line of code to create a simple popup menu button with menu items. In this example we will use popup menu in app bar of our app.
void main() {runApp(const MyApp());}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Popup Menu Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage());
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Popup Menu Demo'),
actions: [
itemBuilder: (context) => [
const PopupMenuItem(child: Text('Settings'),value: 1,),
const PopupMenuItem(child: Text('Privacy Policy'),value: 2,),
const PopupMenuItem(child: Text('Logout'),value: 3,),
])
],
),
);
}
}
Output
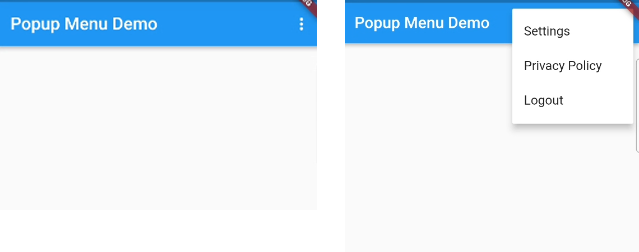
Let’s discuss the above code.
PopupMenuButton(
onSelected: (value){
if(value == 1){
// open settings screen on click on settings
}
},
itemBuilder: (context) => [
const PopupMenuItem(child: Text('Settings'),value: 1,),
const PopupMenuItem(child: Text('Privacy Policy'),value: 2,),
const PopupMenuItem(child: Text('Logout'),value: 3,),
])
This is the main piece of code that crated the popup menu with three menu options, which are called PopupMenuItem. PopupMenuItem is a single menu item in PopupMenuButton. ItemBuilder is a required parameter. We used PopupMenuButton in AppBar using action property. AppBar actions[] property is an array that accepts a list of widgets.
onSelected property is used to perform action on click on any menu item. This property invokes the callback when user selects any option.
Change Icon of Flutter PopupMenuButton.
Default icon of Popup Menu Button is three vertical dots. Flutter provides the flexibility of changing this icon. To change this icon we use the icon property of PopupMenuButton’s constructor.
PopupMenuButton(
icon: const Icon(Icons.more_rounded),
itemBuilder: (context) => [
const PopupMenuItem(child: Text('Settings'),value: 1,),
const PopupMenuItem(child: Text('Privacy Policy'),value: 2,),
const PopupMenuItem(child: Text('Logout'),value: 3,),
])
Output:

Use Text instead of Icon in Flutter PopupMenuButton.
Text can also be used instead of an icon in PopupMenuButton. For this we will use the child property of PopupMenuButton.
PopupMenuButton(
child: const Align(
alignment: Alignment.centerRight,
child: Padding(
padding: EdgeInsets.all(16.0),
child: Text('Menu'),
),
),
itemBuilder: (context) => [
const PopupMenuItem(child: Text('Settings'),value: 1,),
const PopupMenuItem(child: Text('Privacy Policy'),value: 2,),
const PopupMenuItem(child: Text('Logout'),value: 3,),
])
Output:

Change Color of Flutter PopupMenuButton
The color of PopupMenuButton can be changed using color property of PopupMenuButton.
PopupMenuButton(
color: Colors.yellow,
itemBuilder: (context) => [
const PopupMenuItem(child: Text('Settings'),value: 1,),
const PopupMenuItem(child: Text('Privacy Policy'),value: 2,),
const PopupMenuItem(child: Text('Logout'),value: 3,),
])
Output:

Summary
This was the brief detail of FlutterPopupButton. It is an easiest and most used widget in flutter. It is used mostly in app bar and other most commonly used example is to use this in list items. Where each item has multiple options to perform, but there is not extra space to show all options. So this is the best option to show multiples actions on each item of list.