How to show Date Picker on click on TextField in Flutter
It is the most common practice to pick a date by User in a form to save some data. For example you are asking Date of Birth from you user. He has to pick date using date picker. Mostly in this scenario date picker is popped up when user taps on input Field. I this tutorial we will learn how to show Date Picker dialog in Flutter App, on click on TextField.
Here is the example of show Date Picker in Flutter.
DateTime? pickedDate = await showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(1950),
lastDate: DateTime(2050));
This is the simple code to show Date picker dialog. This will prompt a dialog in front of the screen to pick a date. And user can pick date from this dialog.
After picking a desired date, now the next task is to show the user selected date in input filed, which is a TextFormField in Flutter. To do this we need a textInputController for our TextFormField. Here is the full code.
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
TextEditingController dateInputController = TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Dialog'),
),
body: Center(
child: Padding(
padding: const EdgeInsets.symmetric(vertical: 0, horizontal: 20),
child: TextFormField(
decoration: const InputDecoration(
hintText: 'Date',
border: OutlineInputBorder(
borderSide: BorderSide(color: Colors.blue, width: 1)),
focusedBorder: OutlineInputBorder(
borderSide: BorderSide(color: Colors.blue, width: 1)),
enabledBorder: OutlineInputBorder(
borderSide: BorderSide(color: Colors.blue, width: 1)),
),
controller: dateInputController,
readOnly: true,
onTap: () async {
DateTime? pickedDate = await showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(1950),
lastDate: DateTime(2050));
if (pickedDate != null) {
dateInputController.text =pickedDate.toString();
}
},
),
)),
);
}
}
Output

Let’s explore the above code.
How to add border on TextFormFiled?
Here you can see that We created a TextFormField in the center of screen. TextFormField is decorated with blue border. To do this we have user decoration property of TextFormField, and set border, focusedBorder and enabledBorder.
We create the TextFormField read only. So that user cannot edit its content. When user will click on it, date picker dialog will appear. Because we implemented onTap property of TextFormField. When user selects the date, next we show this selected date in TextFormField. This selected date format is not good, There are extra figures in it. To make this date readable we will format this date. To do this we have to use a dart package.
How to format date in Flutter?
Add intl:0.17.0 package in pubspec.yaml file and run this commant in terminal.
Flutter pub get
After that modify code where we have assigned selected date to TextInputController.
if (pickedDate != null) {
dateInputController.text =
DateFormat('dd MMMM yyyy').format(pickedDate);
}
Here we formatted the date in readable form. Now to output will be like this

This is the simple and default date picker dialog of Flutter. Which will open the OS specific date picker in each device. We can also show same date picker dialog in all devices. To do this we have to use dart package in our project. This dart package will show same date picker in all devices regardless of OS.
Use date picker package
Add flutter_holo_date_picker:1.0.3 in pubspec.yaml file and run the flutter pub get command in terminal. Now you can use this date picker in your app. And now in every device you will see the same date picker.
Just update your code of onTap() method
onTap: () async {
var pickedDate = await DatePicker.showSimpleDatePicker(
context,
initialDate: DateTime(1994),
firstDate: DateTime(1960),
lastDate: DateTime(2012),
dateFormat: "dd-MMMM-yyyy",
locale: DateTimePickerLocale.en_us,
looping: true,
);
if (pickedDate != null) {
dateInputController.text =
DateFormat('dd MMMM yyyy').format(pickedDate);
}
},
The date picker will look like this
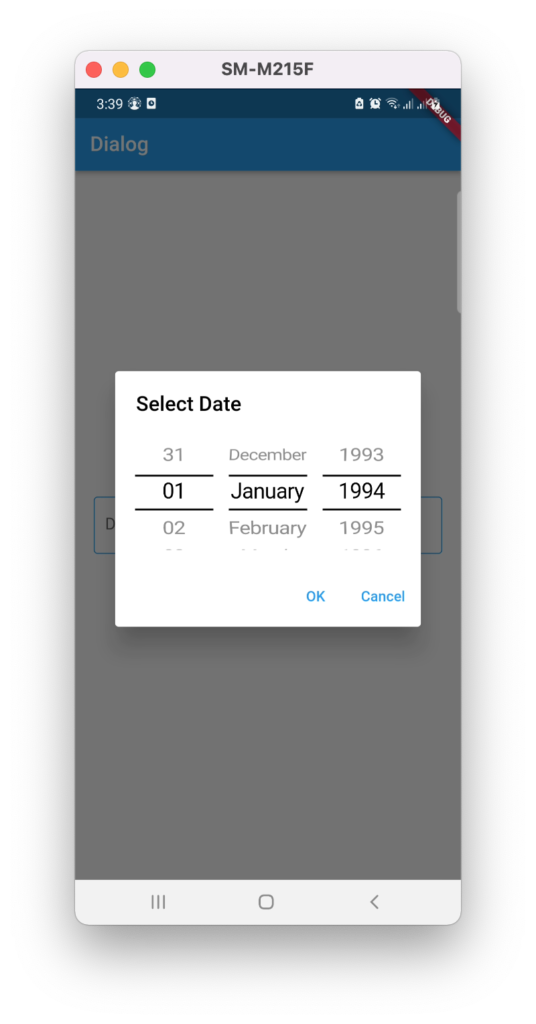
How to show date picker in Flutter Web?
Here we have learned how to show date picker in mobile device. You can use same date pickers in web also. But this is not a good practice. As you know that the user experience of Web is quite different form mobiles. So to select date form user on the web we will use the web date picker package
Add web_date_picker: ^1.0.0+3 this package in pubspec.yaml file and run flutter pub get command in terminal.
Full Code
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
TextEditingController dateInputController = TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Web Date Picker'),
),
body: Center(
child: Padding(
padding: const EdgeInsets.symmetric(vertical: 0, horizontal: 20),
child: WebDatePicker(
onChange: (value) {},
))),
);
}
}
Output
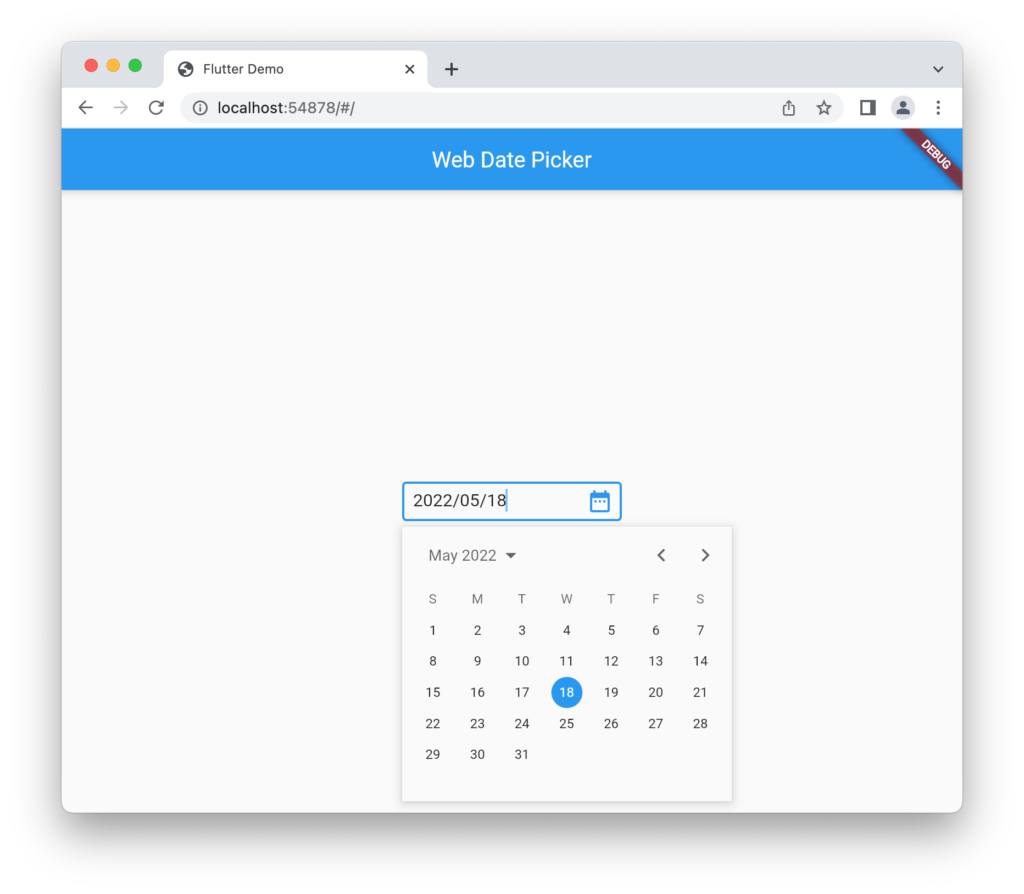
This is the web date picker Which is quite different from mobile’s date picker dialogs. Use this in web Apps instead of default dialogs for better user experience.
Conclusion
In this tutorial we have learned how to show date picker dialog in mobile on click on text filed. And learned how to use date picker dart packages in our Apps to use same date pickers in each device. And we also see the web date picker input filed for better user experience on web. Hope this tutorial will help you in your future projects. Thank you for reading…