How to Check Internet connectivity status in android
Today we are going check internet connectivity status in android programmatically. We will check network status whether it is connected or not. And if internet is connected then what is the type of that network, either it is Wifi or Mobile.
We will check this internet status on clicking on a button and display the network type in TextView. To check the network status we will use ConnectivityManager class.
Check Internet Connectivity Status in Android
Now let’s start this.
Step 1 : Add permissions in manifest file
First of all we need to add network permissions in manifest file.
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
Step 2 : Create an instance of ConnectivityManager
Now we are going to check the status of internet. To check this status, first we will create an instance of ConnectivityManager class like this
val connectivityManager =
context.getSystemService(Context.CONNECTIVITY_SERVICE) as ConnectivityManager
Step 3 : Get Network info from ConnectivityManager
We have created an instance of connectivityManager. This instance will provide us the network info. We will use this info to check the internet connectivity status.
val networkInfo = connectivityManager.activeNetworkInfo
Step 4: Check Network Connection Type
Now we have extracted the network info and this is the time to check the status of internet. We will use instance of network info to check internet connection and type of connection.
if (networkInfo != null) {
if (networkInfo.type == ConnectivityManager.TYPE_WIFI) {
textView.text = "Connected With WIFI"
}
if (networkInfo.type == ConnectivityManager.TYPE_MOBILE) {
textView.text = "Connected With Mobile Network"
}
}
Here we checked that if network info object is not null, it means that internet is connected to mobile. Next we check either the connected network is Wifi of Mobile network and display the name of connected network.
Also read How to check connection status in Flutter
Output
Connected with Mobile Network
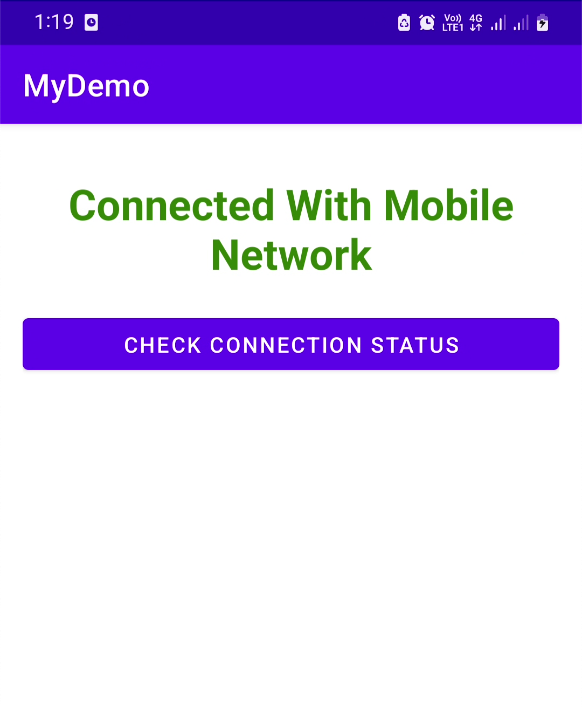
Connected with Wif
