Set hint of Dropdown Button Flutter
Setting a hint of a Flutter DropdownButton is a common practice in user interface design. A hint provides instructional text inside the DropdownButton when no item is selected. It helps users understand the purpose of the dropdown and encourages them to interact with it. For example, a hint like “Select an option” informs users about the expected action.
In situations where the dropdown represents an optional choice, a hint clarifies that selecting an item is not mandatory. This reduces confusion and prevents users from feeling obligated to choose an option when it’s not necessary.
When the widget is first displayed, no item is selected. A hint provides context about what kind of information the dropdown contains. It gives users a visual clue about the type of data they can expect in the dropdown, even before they interact with it.
Let’s see how to set hint of dropdown button in flutter.
Hint of Dropdown Button Flutter
DropdownButton class requires a parameter ‘hint’. This parameter is of widget type. We need to pass Text as parameter.
Code
int? selectedItem;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Dropdown'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Align(
alignment: Alignment.topCenter,
child: DropdownButton(
value: selectedItem,
hint: const Text('Select Option'),
items: const [
DropdownMenuItem(
child: Text('Option A'),
value: 1,
),
DropdownMenuItem(
child: Text('Option B'),
value: 2,
),
],
onChanged: (item) {
setState(() {
selectedItem = item as int;
});
}),
),
),
);
}
Output
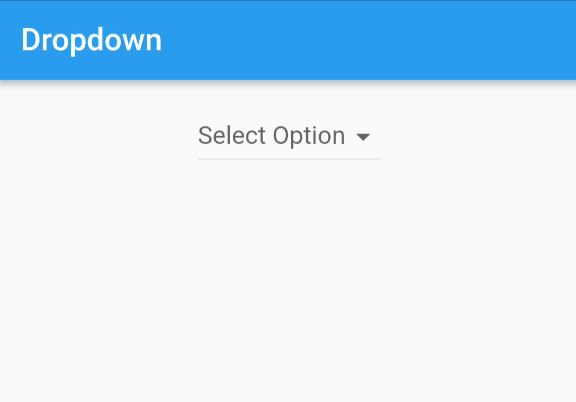
You can also read 2 Ways to add Icon on left of DropdownButton