How to use Grid View in Flutter
Grid view is a graphical element in flutter that is used to display widgets in tabular form. Gridview widget displays its children in two-dimensional array (Columns and rows). As the name demonstrate that we we used it when we want to display items in grid or tabular form. Grid view is a way to show items as grid that comes one after another and below.
Its is like a list as it is scrollable like that. But it is also different form normal list, because list can render elements only in one direction but grid view can render its elements to both horizontal and vertical sides.
We can use grid view in different ways, which are listed below.
- GridView.count
- GridView.builder
- GridView.custom
- GridView.extent
Let us explain all of above mentioned.
GridView.count()
If we know the size of our elements of grid that how many elements we have to show in our girdview, then we will prefer Gridview.count(). It will be a better option for us. GridView.count() is most commonly used as compared to others in flutter.
Let us see an example of this.
A products list is a real time example of GridView. Mostly apps uses Grid View to show their products. We will also show products in GridView in this example. First of all we will create a model class of product.
Create a product.dart file in lib folder, and create a class Product in it.
class Product {
int id;
String name;
String image;
Product(this.id, this.name, this.image);
}
We created a class with three properties, ( id, name and image). We will display product image and name in Gridview. Next we will populate this list with data. For this we override the initstate methond in MyHomePage class which is in main.dart file.
@override
void initState() {
initProductsList();
}
void initProductsList() {
for (int i = 1; i < 21; i++) {
products.add(Product(i, 'product ' + i.toString(),
'https://truth-events.com/wp-content/uploads/2019/09/dummy.jpg'));
}
}
When the flutter starts the screen its initState method is called automatically. So this method will populate our products list. We have added 20 products in list using for loop. Each product has different id, different name with count, and same dummy network image. Now our list of product is populated and is ready for display in GridView.
Now let us see how to display this list. For this go to MyHomePage class and paste this code.
Complete code of home page
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
List<Product> products = [];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('List'),
),
body: GridView.count(
mainAxisSpacing: 4,
crossAxisSpacing: 4,
padding: EdgeInsets.all(8),
crossAxisCount: 3,
children: products
.map((e) => Column(
children: [
SizedBox(
height: 80,
child: Image.network(e.image)),
Text(e.name.toString())
],
))
.toList(),
));
}
@override
void initState() {
initProductsList();
}
void initProductsList() {
for (int i = 1; i < 21; i++) {
products.add(Product(i, 'product ' + i.toString(),
'https://truth-events.com/wp-content/uploads/2019/09/dummy.jpg'));
}
}
}
This is the home screen of our flutter App. Here we used GridView.count() as body of scaffold widget. There are important parameters of GridView.cout(). Let us discuss about these.
First parameter is mainAxisSpacing this is used to add space between the child widgets of main axis.
Second parameter is crossAxisSpacing this is used to add space between the child widgets of cross axis.
Third parameter is padding this is used to add padding between each child widget of Grid View.
Fourth parameter is crossAxisCount this is used to specify the number of columns in Grid View. This will tell that how many widgets will appear in single row of Grid View.
Fifth and most important parameter is children this is used to populate the grid view with data list. This will specify the number of elements in grid view. As we have a list of 20 products, so this grid view will show 20 elements in it with 2 elements in each row.
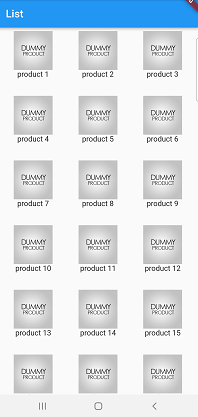