Check internet connection in flutter
In mobile applications, we often need to check internet connection. In this tutorial we are going to check internet connection in flutter application.
Checking the internet connection status in mobile apps is essential to ensure that the app can function properly and provide a seamless user experience. It helps in determining whether the device is connected to the internet and, if so, what type of connection is available (e.g., Wi-Fi, cellular data).
This information allows the app to make decisions about whether to fetch data from remote servers, display content, or enable certain features. It also helps in handling situations when the connection is weak or lost, allowing the app to provide appropriate feedback or switch to offline mode if necessary, ensuring a smoother and more reliable user experience.
How to check internet connection in Flutter
To check internet connection in flutter we will use connectivity_plus plugin. This plugin is designed for checking the status of internet connectivity in flutter application. It provides the simple methods to check connectivity of internet.
Let’s start
Step 1: Add connectivity_plus plugin in pubspec.yaml
Open pubspec.yaml file and add connectivity plus plugin under the dependency section like this.
dependencies:
flutter:
sdk: flutter
connectivity_plus: ^4.0.2
After add this plugin, run the command ‘flutter pub get’i n terminal.
Step 2 : Import connectivity package in dart file
import 'package:connectivity_plus/connectivity_plus.dart';
Step 3 : Check internet connection status
Now we are going to check network connectivity status. To do this we will create a button, and on click on this button we will check the status of internet connection.
Create a string variable ‘connectivityStatus’ that will hold the name of current connected network.
String connectivityStatus = '';
Now create an ElevatedButton and on click on this button, check the status of network connectivity.
ElevatedButton(
onPressed: () async {
final connectivityResult =
await (Connectivity().checkConnectivity());
if (connectivityResult == ConnectivityResult.mobile) {
setState(() {
connectivityStatus = 'Mobile Network Connected';
});
} else if (connectivityResult == ConnectivityResult.wifi) {
setState(() {
connectivityStatus = 'Wifi Connected';
});
}
},
child: const Text('Check Internet Connection')),
Here we are checking the connectivity status of internet, whether it is connected with Wifi or with Mobile Network. And assigning the name of connected network name to connectivityStatus variable.
Step 4: Display connected Network Name
Now we will display the connected network name. To do so we will display a Text Widget and will pass connectivityStatus variable as parameter of Text. It will display the name of connected network .
Here is the full code of this demonstration.
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key});
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
String connectivityStatus = '';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text('Demo'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Center(
child: Column(
children: [
const SizedBox(
height: 20,
),
Text(
connectivityStatus,
style: const TextStyle(
color: Colors.green,
fontSize: 25,
fontWeight: FontWeight.bold,
fontStyle: FontStyle.italic),
),
const SizedBox(
height: 20,
),
ElevatedButton(
onPressed: () async {
final connectivityResult =
await (Connectivity().checkConnectivity());
if (connectivityResult == ConnectivityResult.mobile) {
setState(() {
connectivityStatus = 'Mobile Network Connected';
});
} else if (connectivityResult == ConnectivityResult.wifi) {
setState(() {
connectivityStatus = 'Wifi Connected';
});
}
},
child: const Text('Check Internet Connection')),
],
),
),
),
);
}
}
Output
Connected with wifi
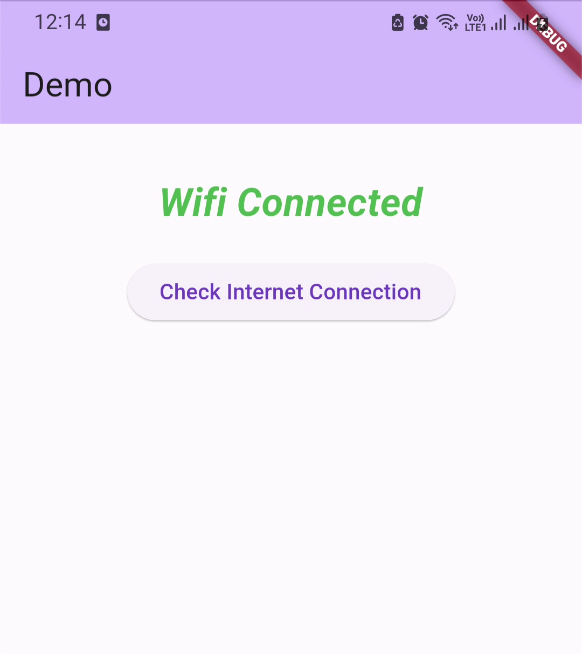
Connected with Mobile Network
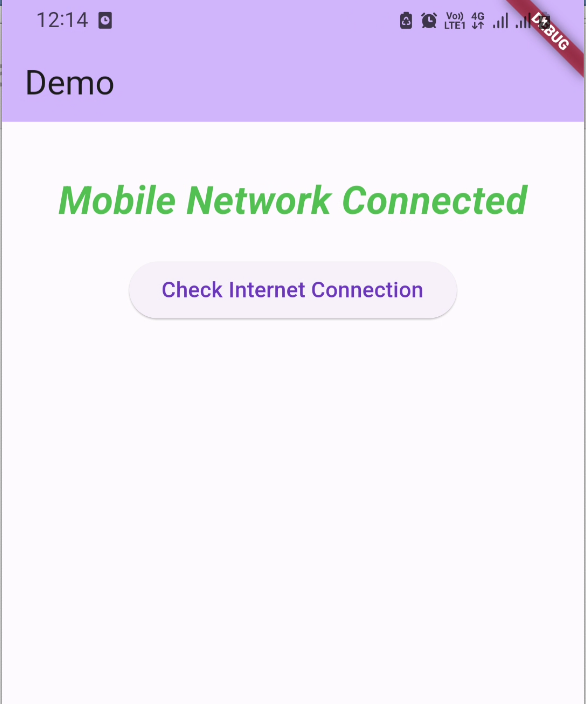