Show date picker dialog in kotlin in android
In this tutorial, we will demonstrate how to implement a date picker dialog in an Android application using the Kotlin programming language. We’ll begin by creating an EditText widget. Upon clicking this EditText, we will display a date picker dialog to choose a date. After selecting the date from the dialog, we will populate the chosen date into the EditText field to display the selected date.
Let’s do it.
Step 1:
Create a EditText in activity_main.xml file.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
tools:context=".MainActivity">
<EditText
android:focusable="false"
android:id="@+id/edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_centerInParent="true" />
</RelativeLayout>
Step 2 :
Access EditText widget in kotlin file
val editText: EditText = findViewById(R.id.edit_text)
Step 3 :
Create a date listener that we will be use in date picker dialog.
var cal = Calendar.getInstance()
val dateSetListener =
DatePickerDialog.OnDateSetListener { view, year, monthOfYear, dayOfMonth ->
cal.set(Calendar.YEAR, year)
cal.set(Calendar.MONTH, monthOfYear)
cal.set(Calendar.DAY_OF_MONTH, dayOfMonth)
val myFormat = "dd MMM yyyy" // mention the format you need
val sdf = SimpleDateFormat(myFormat, Locale.US)
editText.setText(sdf.format(cal.time))
}
step 4 :
Set click listener on EditText and show date picker dialog on click on it.
DatePickerDialog(
this@MainActivity, dateSetListener,
cal.get(Calendar.YEAR),
cal.get(Calendar.MONTH),
cal.get(Calendar.DAY_OF_MONTH)
).show()
Complete Code
package com.app.mydemo
import android.app.DatePickerDialog
import android.os.Bundle
import android.widget.EditText
import androidx.appcompat.app.AppCompatActivity
import java.text.SimpleDateFormat
import java.util.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val editText: EditText = findViewById(R.id.edit_text)
var cal = Calendar.getInstance()
val dateSetListener =
DatePickerDialog.OnDateSetListener { view, year, monthOfYear, dayOfMonth ->
cal.set(Calendar.YEAR, year)
cal.set(Calendar.MONTH, monthOfYear)
cal.set(Calendar.DAY_OF_MONTH, dayOfMonth)
val myFormat = "dd MMM yyyy" // mention the format you need
val sdf = SimpleDateFormat(myFormat, Locale.US)
editText.setText(sdf.format(cal.time))
}
editText.setOnClickListener {
DatePickerDialog(
this@MainActivity, dateSetListener,
cal.get(Calendar.YEAR),
cal.get(Calendar.MONTH),
cal.get(Calendar.DAY_OF_MONTH)
).show()
}
}
}
Output
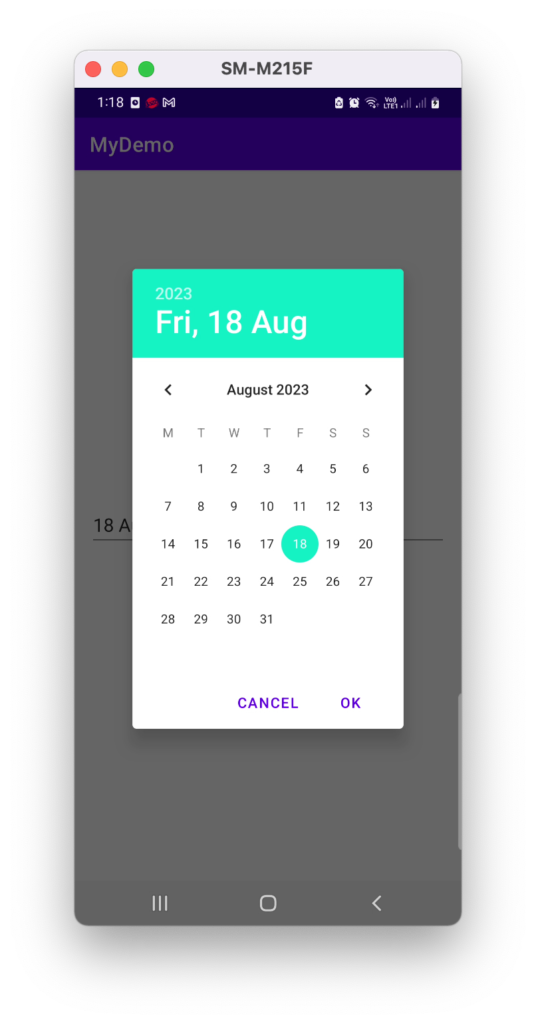
