How to parse json in dart flutter
Obviously json parsing in flutter is not as easy as in android using gson library. Gson has made the json parsing very easy in android. But in flutter json parsing confuses the beginners. In this article we will learn how to parse json in flutter.
For this practice we will use built in library dart:convert. This is the most basic and most recommended library for json parsing in small projects. But if you are working on large projects then you can consider code generator libraries like Json Serializable.
Why Json Parsing
Json parsing in important part of apps that fetch data from internet using Rest APIs. When we call Rest API to fetch data from internet. It returns data in json form. We have to display that data on mobile screen in readable form. To make this data readable we have to parse this json data.
Simple Json Parsing in Flutter
Let’s start with very simple json example. If you worked with Rest APIs before then you would be familiar with this simple json.
{
"id":1,
"first_name":"John",
"last_name":" Jennifer",
"age":34,
"email":"johnjennifer@gmail.com"
}
Parse json using jsonDecode method
Dart provides a built in method “jsonDecode(jsonString)” that converts the json string in Map<String, dynamic> object. This method parses any valid json payload.
Let’s decode above json using this method.
final dataJson =
'{"id":1,"first_name":"John","last_name":"Jennifer","age":34,"email":"johnjennifer@gmail.com"}';
Map<String, dynamic> parsedJson = jsonDecode(dataJson);
Now we have a parsed json object of type Map<String, dynamic>. Because each key of json is type of string but each value of json is of different primitive type. We can easily access all the data of json using this parsed json. For example we want to display the first name. We will retrieve first name like this
Text(parsedJson[‘first_name’]).
This line will show the first name which s “John” in json .
Here is the full code of parsing json using jsonDecode method .
main.dart
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final dataJson =
'{"id":1,"first_name":"John","last_name":"Jennifer","age":34,"email":"johnjennifer@gmail.com"}';
@override
Widget build(BuildContext context) {
Map<String, dynamic> parsedJson = jsonDecode(dataJson);
return Scaffold(
appBar: AppBar(
title: const Text('Json parsing demo'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
children: <Widget>[
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('First Name'),
Text(parsedJson['first_name'],style: const TextStyle(fontWeight: FontWeight.bold),)
],
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('Last Name'),
Text(parsedJson['last_name'],style: const TextStyle(fontWeight: FontWeight.bold),)
],
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('Age'),
Text(parsedJson['age'].toString(),style: const TextStyle(fontWeight: FontWeight.bold),),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('Email'),
Text(parsedJson['email'],style: const TextStyle(fontWeight: FontWeight.bold),)
],
),
],
),
),
);
}
}
Output
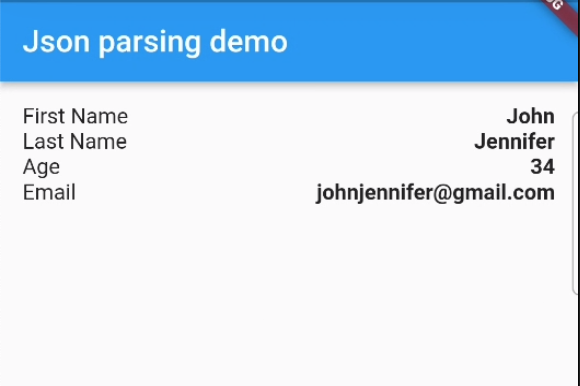
This is the very simple and basic parsing method of json in Flutter. But this is not the type-safety. Using this method we cannot take advantages of strong type-safety of dart language. Because json contains values regardless of any data type. A much better approach is to create a model class in dart and parse json data into that model class.
Parse Json to Dart Model Class – Flutter
Let us create a dart model class for above json. As you see that json contains information about user like his id, first name, last name, age and email. So we will create these properties in dart model class.
Create a dart file named user.dart, and paste this code there.
class User {
int id;
String firstName;
String lastName;
int age;
String email;
User(
{required this.id,
required this.firstName,
required this.lastName,
required this.age,
required this.email});
factory User.fromJson(Map<String, dynamic> json) {
return User(
id: json['id'],
firstName: json['first_name'],
lastName: json['last_name'],
age: json['age'],
email: json['email']);
}
}
Here we take advantage of factory constructor. Now rather than reading data like this.
parsedJson['first_name'];
parsedJson['last_name'];
We can read it like this.
user.firstName;
user.lastName;
This is much clean code and can be parse json to model class easily like this.
Map<String, dynamic> parsedJson = jsonDecode(dataJson);
User user = User.fromJson(parsedJson);
Here is the full code of parsing json to dart model class
main.dart
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final dataJson =
'{"id":1,"first_name":"John","last_name":"Jennifer","age":34,"email":"johnjennifer@gmail.com"}';
@override
Widget build(BuildContext context) {
Map<String, dynamic> parsedJson = jsonDecode(dataJson);
User user = User.fromJson(parsedJson);
return Scaffold(
appBar: AppBar(
title: const Text('Json parsing demo'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
children: <Widget>[
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('First Name'),
Text(
user.firstName,
style: const TextStyle(fontWeight: FontWeight.bold),
)
],
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('Last Name'),
Text(
user.lastName,
style: const TextStyle(fontWeight: FontWeight.bold),
)
],
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('Age'),
Text(
user.age.toString(),
style: const TextStyle(fontWeight: FontWeight.bold),
),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('Email'),
Text(
user.email,
style: const TextStyle(fontWeight: FontWeight.bold),
)
],
),
],
),
),
);
}
}
Here you can see that we just replaced the data reading method from parsedJson[‘first_name’]; to user.firstName for all fields.
This is the simple json parsing. In the next tutorial we will learn how to parse complex json in flutter dart.