Show time picker dialog in Flutter
Today, we will be demonstrating how to display a time picker dialog upon clicking a TextField in a Flutter app. When the TextField is tapped, a time picker dialog will appear, allowing the user to select a time. The chosen time will then be formatted and displayed within the TextField.
Step 1:
To start, let’s create a TextField in Flutter. We’ll pass a TextEditingController to it and then disable the TextField. This is because the TextField should not be focused, and user input should not be allowed.
TextField(
enabled: false,
controller: timeTextController,
)
Step 2:
Let’s proceed to wrap the TextField with an InkWell widget. This will allow us to click on it and display the TimePicker Dialog.
InkWell(
onTap: () async {
},
child: TextField(
enabled: false,
controller: timeTextController,
),
)
Step 3 :
Now on click on TextField we will display TimePicker dialog.
Code to show TimePickerDialog
await showTimePicker(
context: context, initialTime: TimeOfDay.now());
This code will show TimePicker dialog. Write this code in onTap callback of Inkwell.
InkWell(
onTap: () async {
TimeOfDay? pickedTime = await showTimePicker(
context: context, initialTime: TimeOfDay.now());
},
child: TextField(
enabled: false,
controller: timeTextController,
),
)
Step 4:
Now we need to show the selected time in TextField.
InkWell(
onTap: () async {
TimeOfDay? pickedTime = await showTimePicker(
context: context, initialTime: TimeOfDay.now());
timeTextController.text = pickedTime!.format(context).toString();
},
child: TextField(
enabled: false,
controller: timeTextController,
),
)
Output:
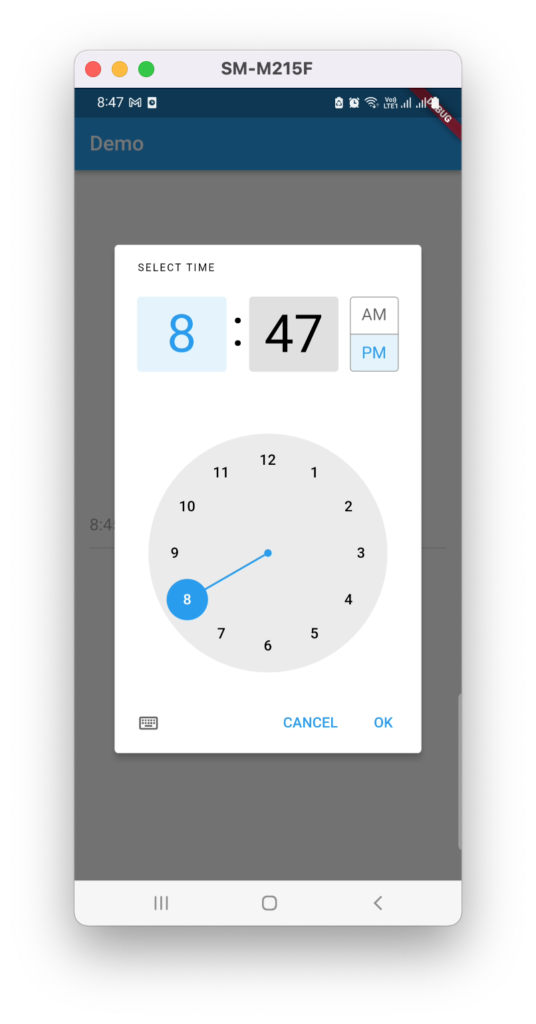
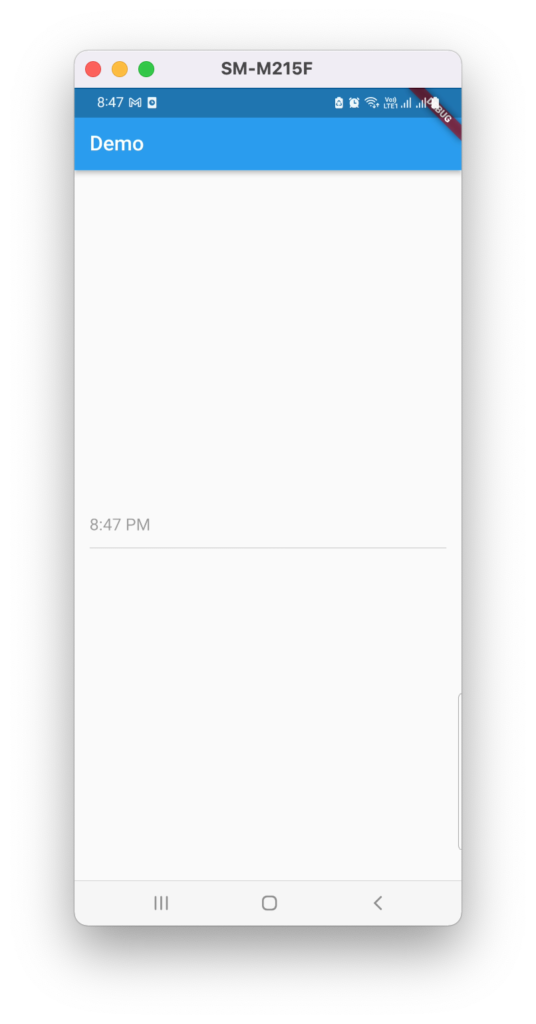